Could anyone explain to me the difference between these two below probes?
out_p = nengo.Probe(out, label="out_p")
out_p_filt = nengo.Probe(out, synapse=0.1, label="out_p_filt")
In the document was told that one without a filter and the other with the filter but I didn’t understand very well.
Is the synapse parameter the same as synapse weight?
Hello @arminsoltan, welcome to our community!
synapse
parameter is used for smoothing the input/output signal; and the signal can be anything i.e. a continuous or discrete signal (e.g. spikes which are discrete). In the code below I have tried to explain the effect of synapse
parameter.
import nengo
import matplotlib.pyplot as plt
# `spike_generator()` generates spikes of amplitude 10.0 every 5th timestep.
def spike_generator(t, freq=5):
if (int(t*1000.0) % freq == 0): # Note: `t` starts at 0.001.
return 10.0
else:
return 0.0
# Create the Nengo Network.
with nengo.Network() as net:
sg = nengo.Node(output=spike_generator, size_out=1)
probe1 = nengo.Probe(sg, synapse=None) # Unfiltered Spikes.
probe2 = nengo.Probe(sg, synapse=0.005) # Filtered Spikes (i.e. somewhat smoothed spikes)
with nengo.Simulator(net) as sim:
sim.run(0.2) # Run it for 200 timesteps.
# Plot the probe results.
ax1 = plt.subplot(2, 1, 1)
ax1.set_title("Un-Filtered Spikes")
ax1.plot(sim.data[probe1])
ax2 = plt.subplot(2, 1, 2)
ax2.set_title("Filtered Spikes")
ax2.plot(sim.data[probe2])
plt.tight_layout()
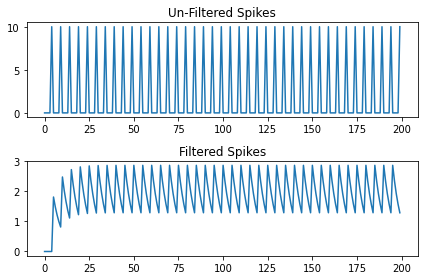
As you can see above, on plotting the probe2
results, we get a somewhat smoothed signal compared to the output of probe1
results (where the synapse
is set to None
). Try playing with the synapse
parameter in line probe2 = nengo.Probe(sg, synapse=0.005)
by setting it to different values i.e. 0.1, 0.001 and so on… to see its effects.
More technically, synapse
parameter applies a synaptic filtering with a default Low Pass filter (i.e. nengo.Lowpass()
. More info here. It is not the same as synaptic weight. You rather set the connection “weights” via the transform
parameter of nengo.Connection()
(more info here).
2 Likes